Archive for March, 2010
Brief Hints: C# Nullable Types and Arrays, Special Double Values
Brief Hints: I wanted to show you three things in C# that I’ve been using a lot lately.
Nullable Types are a convenient language construction in C# that allows one to assign a primitive type with null…
double? someval = null; // declares a nullable double called 'someval'.
The question mark suffixing the keyword double makes the variable someval nullable. This was originally designed so that one can retrieve values from LINQ to SQL without checking for nulls (SQL inherently makes this distinction). This could be thought of as yet another construct to make autoboxing primitives more intuitive and more entrenched in the language.
I use nullable types when I need a special ‘unassigned’ value for “find the greatest” or “find the least” kinds of loops.
When we apply Nullable Types to Arrays, we get an array of nullable primitives (arrays are already nullable, being first class objects).
double?[] somevals = new double?[10]; // declares an array called 'somevals' of ten nullable doubles.
An array of primitives is initialized with all values 0.0; whereas an array of primitve?s is initialized with nulls.
Special Double Values are also something that I’ve started using a lot. There are many algorithms I’ve been coming across that use “magic numbers” corresponding to arbitrarily high and arbitrarily low numbers. Instead of using evil magical quantities, I’ve been using Double.PositiveInfinity and Double.NegativeInfinity. C# makes it easy to assign three more special quantities: Double.NaN, Double.MinValue and Double.MaxValue.
Edit: I forgot to mention why I kept italicizing the word primitive. C# doesn’t really have primitives that are exposed to the developer– everything actually IS an object, and the illusion of boxing or not isn’t really relevant. This just makes nullable types all the more logical.
An Old Physiology Project — Operation Spinny Chair :D
I discovered this ancient report in my repository about three months ago– I’ve finally decided to put it up because it made my day reading the abstract again. This is definitely one of my prouder albeit sillier projects from the days of my undergrad.
Independent Research Project Acute centripetal acceleration is correlated with increased heart rate and R-wave amplitude
Matthew Boyle, Bryan Chung, Eddie Ma
Abstract
In the present study, we set out to discover the correlation between the exposure of acute centripetal acceleration in human subjects and cardiovascular function across the following three dimensions: Heart rate, R-wave amplitude and QRS interval. This was accomplished by measuring the above properties via Lead II Bipolar ECG trace, after having spun the subject at 0.8 revolutions per second in an office chair for successively, 30, 60 and 90 seconds. It was determined that heart rate showed strong positive correlation (n = 3, average increase between trials of increasing duration, 3.2 beats per minute, s = 1.8). R-wave amplitude showed positive correlation in all subjects up until and including the 60 second trial. There was no systemic correlation between duration of spin and the length of the QRS interval in any of the subjects. The heart is therefore an important effector in response to centripetal acceleration in the human model.
Key Words: electrocardiogram, QRS interval, centripetal force, R-wave amplitude, spinning office chair.
[ Download this | PDF ].Thumbnails of pages 5, 7 and 11.
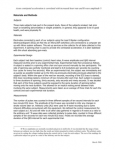
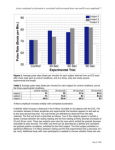
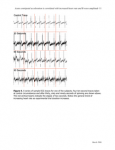
Idea: Delaunay Simplex Graph Grammar
The Structural Bioinformatics course I’m auditing comes with an independent project for graduate students. I’ve decided to see how feasible and meaningful it is to create a graph rewriting grammar for proteins that have been re-expressed as a Delauney Tessellation.
I was first introduced to the Delauney Tessellation about half a year ago. Such a tessellation is composed of irregular three dimensional tetrahedrons where each vertex corresponds to an amino acid. A hypothetical sphere that is defined by the four points of such a tetrahedron cannot be crossed by a line segment that does not belong to said tetrahedron.
An alphabet in formal languages is a finite set of arbitrarily irreducible tokens that composes the inputs of a language. In this project, I want to see if I can discover a grammar for the language of Delauney protein simplex graphs. Graph rewriting is likened to the collapse of neighbouring tetrahedrons. The tetrahedrons selected are either functionally important, stability important or have a strangely high probability of occurrence. This definition is recursively applied so that previously collapsed points are subject to further collapse in future passes of the algorithm.
When a subgraph is rewritten, two things happen. Some meaning is lost from the original representation of the protein, but that same meaning is captured on a stack of the changes made to the representation. In this way, the protein graph is iteratively simplified, while a stack that records the simplifications indicates all of the salient grammatical productions that have been used.
This stack is what my project is really after. Can a stack based on grammatical production rules for frequency of occurrence render any real information, or is it just noise? I can’t even create a solid angle to drive my hypothesis at this point. … “Yes … ?” …
I’ve seen a lot of weird machine learning algorithms in my line of work… and I attest that it’s hard for a novice to look at a description and decide whether or not it derives anything useful. Keep in mind that the literature is chuck full of things that DO work, and none of the things that didn’t make it. I conjecture that this representation has made me optimistically biased.
This method however IS feasible to deploy on short notice in the scope of an independent project 😀