Archive for the ‘Computational Biology’ Category
My talk at Barcode of Life, Adelaide (2011)
I’ve just finished my presentation in Adelaide. This is the first real biology-heavy conference I’ve been to. Sujeevan has brought me along with the BOLD team from BIO in order to present my work — and more importantly — to acquire some resolution about the barcoding culture and its biological significance. The Consortium for The Barcode of Life (CBOL) co-hosted this event with many biodiversity parties in Australia. Another huge group present was the International Barcode of Life (iBOL) project. The Barcode of Life Conference is held every other year and is attended by researchers interested in the concerted international barcoding effort. I presented my preliminary findings with a data analysis session and had excellent feedback — it’s pretty clear where to go next with my thesis! My talk describes the first steps to automating barcode (contig-like) assembly from ab1 sequencer trace files. This talk describes the present need for automation, trends that we can readily detect in currently assembled data and most importantly — detectable patterns in how human experts perform manual barcode assembly.
The full name of the conference is Fourth International Barcode of Life Conference.
>>> Download: ( pdf: EddieMaBOL2011Adelaide.pdf | zip: EddieMaBOL2011Adelaide.pdf.zip ) <<<
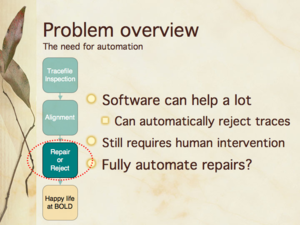
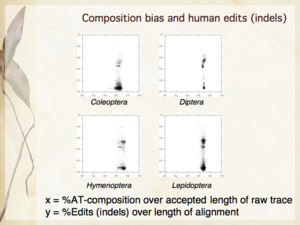
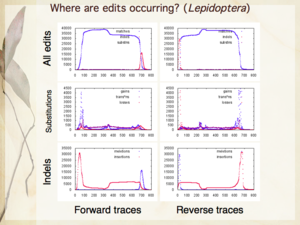
Slides 6, 16, 24 from my presentation — The need for automation; Compositional bias and human edits [null hypothesis]; Where are human edits occurring [in Lepidoptera]?
This has been a very enjoyable conference 😀
C & Bioinformatics: ASCII Nucleotide Comparison Circuit
Here’s a function I developed for Andre about a week ago. The C function takes two arguments. Both arguments are C characters. The first argument corresponds to a degenerate nucleotide as in the below table. The second argument corresponds to a non-degenerate nucleotide {‘A’, ‘C’, ‘G’, ‘T’} or any nucleotide ‘N’. The function returns zero if the logical intersection between the two arguments is zero. The function returns a one-hot binary encoding for the logical intersection if it exists so that {‘A’ = 1, ‘C’ = 2, ‘G’ = 4, ‘T’ = 8} and {‘N’ = 15}. All of this is done treating the lower 5-bits of the ASCII encoding for each character as wires of a circuit.
Character | ASCII (Low 5-Bits) |
Represents | One Hot | Equals |
A |
00001 |
A | 0001 |
1 |
B | 00010 | CGT | 1110 |
14 |
C | 00011 | C | 0010 |
2 |
D | 00100 | AGT | 1101 |
13 |
G | 00111 | G | 0100 |
4 |
H | 01000 | ACT | 1011 |
11 |
K |
01011 | GT | 1100 |
12 |
M | 01101 | AC | 0011 |
3 |
N | 01110 | ACGT | 1111 |
15 |
R | 10010 | AG | 0101 | 5 |
S | 10011 | GC | 0110 |
6 |
T | 10100 | T |
1000 |
8 |
V | 10110 | ACG |
0111 |
7 |
W | 10111 | AT |
1001 |
9 |
Y | 11001 | CT |
1010 |
10 |
The premise is that removing all of the logical branching and using only binary operators would make things a bit faster — I’m actually not sure if the following solution is faster because there are twelve variables local to the function scope — we can be assured that at least half of these variables will be stored outside of cache and will have to live in memory. We’d get a moderate speed boost if at all.
/* f(): Bitwise comparison circuit that treats nucleotide and degenerate nucleotide ascii characters as mathematical sets. The operation performed is set i intersect j. arg char i: Primer -- accepts ascii |ABCDG HKMNR STVWY| = 15. arg char j: Sequence -- accepts ascii |ACGTN| = 5. return char (k = 0): false -- i intersect j is empty. return char (k > 0): 1 -- the intersection is 'A' 2 -- the intersection is 'C' 4 -- the intersection is 'G' 8 -- the intersection is 'T' 15 -- the intersection is 'N' return char (undefined value): ? -- if any other characters are placed in i or j. */ char f(char i, char j) { // break apart Primer into bits ... char p = (i >> 4) &1; char q = (i >> 3) &1; char r = (i >> 2) &1; char s = (i >> 1) &1; char t = i &1; // break apart Sequence into bits ... char a = (j >> 4) &1; char b = (j >> 3) &1; char c = (j >> 2) &1; char d = (j >> 1) &1; char e = j &1; return ( // == PRIMER CIRCUIT == ( // -- A -- ((p|q|r|s )^1) & t | ((p|q| s|t)^1) & r | ((p| r|s|t)^1) & q | ((p| s )^1) & q&r& t | ((p| t)^1) & q&r&s | (( q| t)^1) & p&s | (( q )^1) & p&r&s ) | ( // -- C -- ((p|q|r )^1) & s | ((p| r|s|t)^1) & q | ((p| s )^1) & q&r& t | ((p| t)^1) & q&r&s | (( q|r )^1) & s&t | (( q| t)^1) & p &r&s | (( r|s )^1) & p&q& t ) << 1 | ( // -- G -- (( q|r| t)^1) & s | ((p|q| s|t)^1) & r | ((p|q )^1) & r&s&t | ((p| r )^1) & q& s&t | ((p| t)^1) & q&r&s | (( q|r )^1) & p& s | (( q| t)^1) & p& s ) << 2 | ( // -- T -- ((p|q|r| t)^1) & s | (( q| s|t)^1) & r | ((p| r|s|t)^1) & q | ((p| r )^1) & q& s&t | ((p| t)^1) & q&r&s | (( q )^1) & p& r&s&t | (( r|s )^1) & p&q& t ) << 3 ) & ( // == SEQUENCE CIRCUIT == ( // -- A -- ((a|b|c|d )^1) & e | ((a| e)^1) & b&c&d ) | ( // -- C -- ((a|b|c )^1) & d&e | ((a| e)^1) & b&c&d ) << 1 | ( // -- G -- ((a|b )^1) & c&d&e | ((a| e)^1) & b&c&d ) << 2 | ( // -- T -- ((a| e)^1) & b&c&d | (( b| d|e)^1) & a& c ) << 3 ); }
Andre’s eventual solution was to use a look-up table which very likely proves faster in practice. At the very least, this was a nice refresher and practical example for circuit logic using four sets of minterms (one for each one-hot output wire).
Should you need this logic to build a fast physical circuit or have some magical architecture with a dozen registers (accessible to the compiler), be my guest 😀
Simple Interactive Phylogenetic Tree Sketches JS+SVG+XHTML
>>> Attached: ( parse_newick_xhtml.js | draw_phylogeny.js — implementation in JS | index.html — demo with IL-1 ) <<<
>>> Attached: ( auto_phylo_diagram.tgz — includes all above, a python script, a demo makefile and IL-1 data ) <<<
In this post, I discuss a Python script I wrote that will convert a Newick Traversal and a FASTA file into a browser-viewable interactive diagram using SVG+JS+XHTML. This method is compatible with WebKit (Safari, Chrome) and Mozilla (Firefox) renderers but not Opera. XHTML doesn’t work with IE, so we’ll have to wait for massive adoption of HTML5 before I can make this work for everyone — don’t worry, it’s coming.
To the left is what the rendered tree looks like (shown as a screen captured PNG).
I recommend looking at the demo index.xhtml above if your browser supports it now. The demo will do a far better job than text in explaining exactly what features are possible using this method.
Try clicking on the different nodes and hyperlinks in the demo — notice that the displayed alignment strips away sites (columns) that are 100% gaps so that each node displays only a profile relevant for itself.
I originally intended to explain all the details of the JavaScripts that render and drive the diagram, but I think it’d be more useful to first focus on the Python script and the data input and output from the script.
The Attached Python Script to_xhtml.py found in auto_phylo_diagram.tgz
I’ll explain how to invoke to_xhtml.py with an example below.
python to_xhtml.py IL1fasta.txt IL1tree2.txt "IL-1 group from NCBI CDD (15)" > index.xhtml
Arguments …
- Plain Text FASTA file — IL1fasta.txt
- Plain Text Newick Traversal file — IL1tree2.txt
- Title of the generated XHTML — “IL-1 group from NCBI CDD (15)”
The data I used was generated with MUSCLE with default parameters. I specified the output FASTA with -out and the output second iteration tree with -tree2.
The Attached makefile found in auto_phylo_diagram.tgz
The makefile has two actual rules. If index.html does not exist, it will be regenerated with the above Python script and IL1fasta.txt and IL1tree2.txt. If either IL1fasta.txt or IL1tree2.txt or both do not exist, these files are regenerated using MUSCLE with default parameters on the included example data file ncbi.IL1.cl00094.15.fasta.txt. Running make on the included package shouldn’t do anything since all of the files are included.
The Example Data
The example data comes from the Interleukin-1 group (cd00100) from the NCBI Conserved Domains Database (CDD).
Finally, I’ll discuss briefly the nitty gritty of the JavaScripts found as stand-alones above that are also included in the package.
Part Two of Two
As is the nature of programatically drawing things, this particular task comes with its share of prerequisites.
In a previous — Part 1, I covered Interactive Diagrams Using Inline JS+SVG+XHTML — see that post for compatibility notes and the general method (remember, this technique is a holdover that will eventually be replaced with HTML5 and that the scripts in this post are not compatible with Opera anymore).
In this current post — Part 2, I’ll also assume you’ve seen Parsing a Newick Tree with recursive descent (you don’t need to know it inside and out, since we’ll only use these functions) — if you look at the example index.html attached, you’ll see that we actually parse the Newick Traversal using two functions in parse_newick_xhtml.js — tree_builder() then traverse_tree(). The former converts the traversal to an in-memory binary tree while the latter accepts the root node of said tree and produces an array. This array is read in draw_phylogeny.js by fdraw_tree_svg() which does the actual rendering along with attaching all of the dynamic behaviour.
Parameters ( What are we drawing? )
To keep things simple, let’s focus on three requirements: (1) the tree will me sketched top-down with the root on top and its leaves below; (2) the diagram must make good use of the window width and avoid making horizontal scroll bars (unless it’s absolutely necessary); (3) the drawing function must make the diagram compatible with the overall document object model (DOM) so that we can highlight nodes and know what nodes were clicked to show the appropriate data.
Because of the second condition, all nodes in a given depth of the tree will be drawn at the same time. A horizontal repulsion will be applied so that each level will appear to be centre-justified.
General Strategy
Because we are centre-justifying the tree, we will be drawing each level of the tree simultaneously to calculate the offsets we want for each node. We perform this iteratively for each level of depth of the tree in the function fdraw_tree_svg(). Everything that is written dynamically by JavaScript goes to a div with a unique id. All of the SVG goes to id=”tree_display” and all of the textual output including raster images, text and alignments goes to id=”thoughts”. To attach behaviour to hyperlinks, whether it’s within the SVG or using standard anchor tags, the “onclick” property is defined. In this case, we always call the same function “onclick=javascript:select_node()“. This function accepts a single parameter: the integer serial number that has been clicked.
Additional Behaviour — Images and Links to Databases
After I wrote the first drafts of the JavaScripts, I decided to add two more behaviours in the current version. First, if a particular node name has the letters “GI” in it, the script will attempt to hyperlink it with the proceeding serial number against NCBI. Second, if a particular node name has the letters “PDB” in it, the script will attempt to hyperlink the following identifier against RSCB Protein Database and also pull the first thumbnail with an img tag for the 3D protein structure.
Enjoy!
This was done because I wanted to show a custom alignment algorithm to my advisors. In this demo, I’ve stripped away the custom alignment leaving behind a more general yet pleasing visualizer. I hope that the Python script and JavaScripts are useful to you. Enjoy!
C# & Bioinformatics: Edit Strings and Local Alignment Subprofiles
Alternatively: The awful finite string manipulation bug that took me forever to fix.
Update: Code last update 2010 Dec. 12 (branch logic fixed: gave default “else” case highest priority instead).
Every so often, a bug creeps in during the implementation of a utility function that halts an entire project until it can be fixed. I bumped into one that was so insidious — so retrospectively obvious — that I must share it with you. This particular bug has to do with the way Edit Strings behave when you use them to represent changes done during a Local Alignment rather than a Global Alignment.
Those of you who have looked into MUSCLE may have seen the 2004 BMC paper that goes into great detail about its implementation. In it, Dr. Edgar describes Edit Strings (e-strings) — these are integer strings which keep track of the edits performed on a sequence in order to insert gaps in the correct locations corresponding to an alignment.
I’ve previously discussed and explained e-strings and their use in sequence alignment here and also how to convert alignment strings (a recording of site-to-site insertions/deletions/substitutions) to edit strings here.
E-Strings in Local Alignments
E-strings weren’t originally designed with local alignments in mind — but that’s OK. We can make a few adjustments that will let us keep track of gaps the way we intend them. In a global alignment, all tokens (characters) in each of the aligned sequences are considered. In a local alignment, we need to be able to specify two aligned subsequences rather than the alignment of two full sequences. We can add a simple integer offset to indicate the number of positions since the beginning of aligned sequences to specify the start position of each subsequence.
These are two sequences s and t — (to keep things easy to visualize, I’ve just used characters from the English alphabet).
s = DEIJKLMNOPQRSTUVWXYZ t = ABCDEFGHIJKLMNOUVWXY
Assume that the following is the optimal global alignment of s and t.
s(global) = ---DE---IJKLMNOPQRSTUVWXYZ t(global) = ABCDEFGHIJKLMNO-----UVWXY-
The corresponding edit strings for s and t are es and et respectively.
e(s, global) = < -3, 2, -3, 18 > e(t, global) = < 15, -5, 5, -1 >
Remember, we can take the sum of the absolute values in two edit strings corresponding to the same alignment to be sure that they contain the number of sites. This is true because a site in a given sequence or profile must be either an amino acid (in a sequence) or a distribution of amino acids (in a profile) or a gap (in either). In this case, the absolute values of both es and et correctly add up to 26.
Now let’s adapt the above to local alignments.
The corresponding optimal local alignment for s and t is as follows.
s(local) = DE---IJKLMNOPQRSTUVWXY t(local) = DEFGHIJKLMNO-----UVWXY
The edit strings only need an offset to specify the correct start position in each sequence. Remember that our indexing is zero based, so the position for the character “A” was at position zero.
e(s, local) = 3 + < 2, -3, 17 > e(t, local) = 3 + < 12, -5, 5 >
It’s a pretty straight forward fix. Notice that the absolute values of the integers inside the vectors now only sum up to 22 (corresponding to the sites stretching from “D” to “Y”).
Local Alignment E-String Subprofiles
The bug I faced wasn’t in the above however. It only occurred when I needed to specify subprofiles of local alignments. Imagine having a local alignment of two sequences in memory. Imagine now that there is a specific run of aligned sites therein that you are interested in and want to extract. That specific run should be specifiable by using the original sequences and a corresponding pair of e-strings and pair of offsets. Ideally, the new e-strings and offsets should be derived from the e-strings and offsets we had gathered from performing the original local alignment. We call this specific run of aligned sites a subprofile.
As you will see, this bug is actually a result of forgetting to count the number of gaps needed that occur after the original local alignment offset and before where the new subprofile should start. Don’t worry, this will become clearer with an example.
For the local alignment of s and t, I want to specify a subprofile stretching from the site at letter “I” to the site at letter “V”.
s(local) = DE---IJKLMNOPQRSTUVWXY → s(subprofile) = IJKLMNOPQRSTUV t(local) = DEFGHIJKLMNO-----UVWXY → t(subprofile) = IJKLMNO-----UV
What do you suppose the edit string to derive would be?
Let’s puzzle this out — we want to take a substring from each s and t from position 5 to position 18 of the local alignment as shown below …
Position: 0 0 1 2 0 5 8 1 s(local) = DE---IJKLMNOPQRSTUVWXY → s(subprofile) = IJKLMNOPQRSTUV | | | | t(local) = DEFGHIJKLMNO-----UVWXY → t(subprofile) = IJKLMNO-----UV | | |<- Want ->|
Let’s break this down further and get first the vector part of the e-string and then the offset part of the e-string.
Getting the Vector part of the Edit String
Remember, we’re trying to get an edit string that will operate on the original sequences s, t and return ssubprofile, tsubprofile. Getting the vector part of the estring is straight forward, you literally find all of the sites specified in the subsequence and save them. Since we want positions [5, 18] in the local edit strings, we retrieve the parts of the edit strings which correspond to those sites (we’ll ignore offsets for now). To visualize this site-to-site mapping, let’s decompose our local estrings into something I call unit-estrings — an equivalent estring which only uses positive and negative ones (given as “+” and “-” below in the unit vectors us, ut for s, t respectively).
Position: 0 0 1 2 0 5 8 1 s(local) = D E - - - I J K L M N O P Q R S T U V W X Y e(s, vector) = < 2, -3, 17 > -> u(s) = < + + - - - + + + + + + + + + + + + + + + + + > e(s, newvec) = < 14 > <- < + + + + + + + + + + + + + + > |<- want! ->| e(t, newvec) = < 7, -5, 2 > <- < + + + + + + + - - - - - + + > e(t, vector) = < 12, -5, 5 > -> u(t) = < + + + + + + + + + + + + - - - - - + + + + + > t(local) = D E F G H I J K L M N O - - - - - U V W X Y
We retrieve as our new vectors es, newvec = < 14 > and et, newvec = < 7, -5, 2 >. Notice that the integer vector elements are just a count of the runs of positive and negative ones in the subprofile region that we want.
Getting the Offset part of the Edit String (the Right way)
This part gets a bit tricky. The offset is a sum of three items.
- The offset from the original sequence to the local alignment.
- The offset from the local alignment to the subprofile.
- The negative of all of the gap characters that are inserted in the local alignment until the start of the subprofile.
The below diagram shows where each of these values come from.
s = DEIJKLMNOPQRSTUVWXYZ s(local) = DE---IJKLMNOPQRSTUVWXY -> +0 -- offset from original to local s(subprofile) = .....IJKLMNOPQRSTUV -> +5 -- offset from local to subprofile --- -> -3 -- gaps: local start to subprofile start => +2 == TOTAL | | t = ABCDEFGHIJKLMNOUVWXY t(local) = ...DEFGHIJKLMNO-----UVWXY -> +3 -- offset from original to local t(subprofile) = .....IJKLMNO-----UV -> +5 -- offset from local to subprofile -> -0 -- gaps: local start to subprofile start => +8 == TOTAL
You might be wondering about the weird gap subtraction for sequence s. This is the solution to my bug! We need this gap subtraction! When you forget to subtract these gaps, you end up with an alignment that is incorrect where the subprofile of s starts too late. But why is this so? When we transform a sequence from the original to the subprofile, the gaps that we’ve taken for granted in the local alignment don’t exist yet. This way, we would be mistakenly pushing our subprofile into a position where non-existent gapped sites are virtually aligned to existing amino acid sites in the alignment partner t. This was a very odd concept for me to grasp, so let yourself think on this for a bit if it still doesn’t make sense. … You could always take it for granted too once I show you below that this is indeed the correct solution 😛
The completed edit strings to specify the subprofile from “I” to “V” for the local alignment of s and t are thus:
e(s, subprofile) = 2 + < 14 > e(t, subprofile) = 8 + < 7, -5, 2 >
So let’s make sure these work — let’s apply es, subprofile and et, subprofile onto s and t to see if we get back the correct subprofiles.
>>> apply offsets ... s = DEIJKLMNOPQRSTUVWXYZ : +2 remaining ... s = IJKLMNOPQRSTUVWXYZ t = ABCDEFGHIJKLMNOUVWXY : +8 remaining ... t = IJKLMNOUVWXY >>> apply e-strings ... s = IJKLMNOPQRSTUVWXYZ : < 14 > remaining ... s = IJKLMNOPQRSTUV t = IJKLMNOUVWXY : < 7, -5, 2 > remaining ... t = IJKLMNO : UVWXY : < -5, 2 > remaining ... t = IJKLMNO----- : UVWXY : < 2 > remaining ... t = IJKLMNO-----UV s = IJKLMNOPQRSTUV -- selected subprofile ok. t = IJKLMNO-----UV -- selected subprofile ok.
The above application results in the desired subprofile.
The SubEString Function in C#
The below source code gives the correct implementation in order to find the e-string corresponding to a subprofile with the following arguments. It is an extension function for List<int> — a natural choice since I am treating lists of integer type as edit strings in my project.
- this List<int> u — the local alignment e-string from which to create the subprofile e-string.
- int start — the offset from the left specifying the location of the subprofile with respect to the local alignment.
- int end_before — the indexed site before which we stop; this is different from length since start and end_before are zero-based and are specified from the very start of the local alignment.
- out int lostGaps — returns a negative value to add to the offset — this is the number of gaps that occur between the start of the local alignment and the start of the subprofile.
This function returns a new List<int> e-string as you would expect.
public static List<int> SubEString( this List<int> u, int start, int end_before, out int lostGaps) { if(end_before < start) { Console.Error.WriteLine("Error: end_before < start?! WHY?"); throw new ValueOutOfRange(); } var retObj = new List<int>(); var consumed = 0; // the amount "consumed" so far. var remaining_start = start; // subtract "remaining_start" to zero. var ui = 0; // the current index in the e-string var uu = 0; // the current element of the e-string var uu_abs = 0; // the absolute value of the current element lostGaps = 0; // the number of gaps that is subtracted from the offset. //START -- dealing with the "start" argument ... for(; ui < u.Count; ui ++) { uu = u[ui]; uu_abs = Math.Abs(uu); if(uu_abs < remaining_start) { if(uu < 0) lostGaps += uu; //LOSTGAPS // add uu this time // it's the fraction of remaining_start we care about. consumed += uu_abs; // uu smaller than start amount, eat uu. remaining_start -= uu_abs; // start shrinks by uu. uu_abs = 0; // no uu left. uu = 0; // no uu left. // not done -- go back and get more uu for me to eat. } else if(consumed + uu_abs == remaining_start) { if(uu < 0) lostGaps += -remaining_start; //LOSTGAPS // -- if thus uu is equal to the start amount, // count them into LOSTGAPS // we want to get everything occurring before the subestring start consumed += remaining_start; // same size -- consume start amount. uu_abs = 0; // MAD (mutually assured destruction 0 -> 0) remaining_start = 0; // MAD uu = 0; // MAD ui ++; break; // done. } else if(consumed + uu_abs > remaining_start) { if(consumed + uu_abs > end_before && consumed < remaining_start) { // easier to say ui == u.Count-1? if(uu < 0) lostGaps += -remaining_start; //LOSTGAPS uu_abs = (end_before - (consumed + remaining_start)); uu = uu > 0 ? uu_abs : -uu_abs; retObj.Add(uu); return retObj; } else if(consumed + uu_abs < end_before) { consumed += uu_abs; // Seemingly wrong -- however: // push all the way to the end; subtract the beginning of uu_abs // ensures correct calculation of remaining string uu_abs -= remaining_start; // remove only start amount. // we're getting the end half of uu_abs! if(uu < 0) lostGaps += -remaining_start; //LOSTGAPS remaining_start = 0; // no start left. uu = uu > 0 ? uu_abs : -uu_abs; // remaining uu at this ui. if(uu != 0) retObj.Add(uu); // fixes a zero-entry bug ui ++; break; // done. } else { if(uu < 0) lostGaps += -remaining_start; //LOSTGAPS uu_abs = (end_before - remaining_start); uu = uu > 0 ? uu_abs : -uu_abs; retObj.Add(uu); return retObj; } } } //END_BEFORE -- dealing with the "end_before" argument ... for(; ui < u.Count; ui ++) { uu = u[ui]; uu_abs = Math.Abs(uu); if((consumed + uu_abs) == end_before) { retObj.Add(uu); break; } else if((consumed + uu_abs) < end_before) { retObj.Add(uu); consumed += uu_abs; } else /*if((consumed + uu_abs) > end_before)*/ { uu_abs = end_before - consumed; uu = uu > 0 ? uu_abs : -uu_abs; retObj.Add(uu); break; } } return retObj; }
I’ve really sent the above code through unit tests and also in practical deployment so it should be relatively bug free. I hope that by posting this here, you can tease out the logic from both the code and the comments and port it to your preferred language. In retrospect, maybe this bug wasn’t so obvious after all. When you think about it, each of the pieces of code and logic are doing exactly what we asked them to. It was only in chaining together an e-string subprofile function along with a local alignment that this thing ever even popped out. Either way, the fix is here. Hopefully it’ll be helpful.
Two Important Notes
- Since global alignments represent the aligned sites of all sequences involved, no offset is used and hence this bug would never occur when specifying a subprofile of a global alignment.
- Gaps that occur in the original sequence don’t affect retrieving the subprofile e-string calculation at all because those gaps DO exist and can be authentically counted into an offset.
Next
I haven’t had a need yet for porting the multiply function (earlier post) from global alignment logic to local alignment logic. If there are any insidious logical flaws like the ones I encountered this time, I’ll be sure to make a post.
BioCompiler might start life as BioCOBOL
Update: Matthew has found an even more thorough review paper that discusses computer assisted synthetic biology approaches — it can be found at doi:10.1016/j.copbio.2009.08.007.
The iGEM competition year is running to a close. The teams are headed into November 2010 and have roughly one month left before attending the conference. I’m personally not attending the conference this year — I think the undergraduates will get more out of the experience.
The current year sees our continued efforts to precipitate a dedicated software team — a functioning autonomous unit that will serve to supplement and enhance Waterloo’s impact in the iGEM competition. More importantly, we’re going to do some very interesting science. We’ve had some success talking with other student groups across campus — notably, we should probably talk with the student IEEE/CUBE chapter when we have more work completed. We had involved BIC as well, however, it was early on and we had even less ground to stand upon ( — the primordial software team was mostly interested in BioMortar and BrickLayer at the time — the later project having been taken up by the iGEM Coop students as in Python this year).
This whole BioCompiler business started when Matthew uncovered a nice candidate problem: the compilation of a schematic for behaviour to a fully functioning synthetic biological circuit. Let’s be as precise as we can be here. I mean to say, we will take a description (which could resemble a piece of formal language source code) — and have it compiled into the sequence of BioBricks that will produce the desired behaviour.
This idea has been approached by several groups before — but each time, a different subproblem was considered (this is a reorganization of Matthew’s very nice list here).
- Synthetic biology programming language: Genetic Engineering of Living Cells (GEC) (Microsoft Research) is a project that hones in on a formal language specification.
- Synthetic biology computer assisted design (CAD): Berkeley Software (iGEM 2009) created a suite of items — Eugene (formal language for synthetic biology), Spectacles (visualizer integrating parts with their behaviours), Kepler (a dataflow broker). As well, Berkeley is responsible for the award winning Clotho (iGEM 2008 – Best Software Tool) which is a workbench that connects with the parts registry database (amongst other possible resources).
- Systems biology pathway reaction simulation: Systems Biology Markup Language (SBML), Systems Biology Workbench (SBW) and Jarnac are a set of tools that perform systems biology analysis (which we consider to be an output of synthetic biology). SBML is the formal language, Jarnac is a reaction network simulator (which utilizes JDesigner as a front end) and SBML is a dataflow broker between SBML and Jarnac.
- BioBrick specific pathway simulation: Minnesota’s Team Comparator (iGEM 2008) created SynBioSS — a tool which estimates the concentration of reactants and products given the appropriate BioBricks on a simulated circuit.
Update — here are a few more items thanks to Matthew Gingerich, George Zarubin and Andre Masella.
- Molecular biology and bioinformatics analysis: The European Molecular Biology Open Software Suite (EMBOSS) is a toolkit developed by the European Molecular Biology Network (EMBnet) for bioinformatics. This might not be immediately relevant, but it is interesting. EMBOSS is actually relatively complete. More distantly along this vein, there’s also Bioconductor which focuses mostly on microarray analysis and is implemented in R.
- More synthetic biology computer aided design (CAD): TinkerCell is a GUI-driven piece of software that supports extension with C++ and Python. TinkerCell along with the suite created by Berkeley Software are the two most promising target systems in which to integrate BioCompiler. Finally, there’s GenoCAD which appears to be in an early phase of development — this software looks to emphasize construction with correct syntax and attribute grammars — I’ll have to read more about this.
- Formal laboratory protocol description: BioCoder is another Microsoft research project — the designed language aims to be both human readable and complete for automation. It reminds me of standard operating procedures (SOP) with greater precision. While BioCoder compiles from protocol to automation, we’ll be compiling to circuitry and protocol. BioCoder will give us some insights about the kinds of protocols that others are thinking of.
There is of course more software, but these are the items that we have become most familiar with — that we like — and that we consider to be standards toward which our own work should strive.
Two interesting problems arise when we think about these subproblems. First, the programming languages specified (including Eugene from Berkeley’s CAD suite) are exactly what they claim to be. Formal specifications. This is possible because of how concrete they are. They literally document what a synthetic biology circuit is. But this isn’t too different from what humans have been doing in iGEM all along. Second, the synthetic biology items don’t really seem to talk to the systems biology items — whereas we expect that the two — being input and output — to be inextricably linked. I explain my thoughts on the two below.
Why a programming language?
Andre enlightened me to this the other day. Humans invented programming languages to do two things. On an arrow running from the concrete toward the esoteric, we have the practical concern of compressing the amount of code that we want to write while retaining our programs’ expressiveness. This is the origin of macro systems such as COBOL. On an arrow pointing in the reverse — from the cerebral abstract down to the literal — we have the theoretical concern of mathematical beauty, of completeness. This is the origin of such functional languages like Lisp. For humans to have any hope of creating such a Lisp-like language for synthetic biology — we would need to understand all of the reactionary nuances about it, the system with which we tamper — at least inasmuch as a painful heuristic approximation. This is a feat we are no where near completing though footholds are managed with systems biology.
So here we are, BioCOBOL is the first step. A developing simple — though complete macro-like system that is in league in terms of abstraction with the programming language / CAD -like projects we’ve seen thus far. Only, we aim to increase the efficiency of circuit design; so that the programming language is not a literal mapping of the human document — rather, it is an explanation of behaviour. We will abstract it ever so slightly with each iteration of development — departing further and further away from CAD. A subteam headed by Brandon is currently developing the syntax and search algorithms required for the job — Brandon suggests that a weighted traversal of valid circuits should form our algorithmic primitive. My subteam is attempting to characterize as many known circuits in iGEM as possible — analyzing what pieces of input (stimuli: chemical concentrations, gradients, quora, oscillators) may be compressed for their common usage — what function prototypes already exist ( — reacts to an input: promoters) for compression — what the standard outputs are (analogy to printing error messages: GFP-family) etc. — again in the effort to realize what is losslessly compressible. Our software should eventually provide the correct laboratory protocol as well.
In this sense, we are respecting what a compiler is before we even approach more sophisticated compilation: it transforms a document in one language to another.
Incidentally, I should mention that Jordan and George are working on a modelling problem with the lab team — I’m not clear on the specifics, but I take it they want to pull out some differential equations on a set of promoters.
Why do we care that Synthetic Biology logic should talk to Systems Biology logic?
Finally, it is clear that we will eventually want to become even more abstract — even more mathematically complete, even more expressive. While we may never know enough about systems biology to create BioLISP (in our lifetime), we expect there to be sufficient research for us to discover — and perhaps research we can conduct ourselves to come ever closer. Systems biology allows us to think about synthetic biology in terms of reaction concentrations; free energy etc.. It gives the notion of compilation its own ground; the ground we want to cover. Imagine the perfect BioCompiler — stating the a problem to be compiled in terms of the input and output of the system. Let’s be precise here: I mean to say, the products and reactants or behaviour of our circuit. Let us describe what our circuit will do instead of what our circuit is made of. This — the missing link, this compiler — is the logical final step of BioCompiler.
C# & Bioinformatics: Indexers & Substitution Matrices
I’ve recently come to appreciate the convenience of C# indexers. What indexers allow you to do is to subscript an object using the familiar bracket notation. I’ve used them for substitution matrices as part of my phylogeny project. Indexers are essentially syntactical sugar that obey the rules of method overloading. I first describe what I think are useful substitution matrix indexers and then a bare bones substitution matrix class (you could use your own). The indexer notation implementation is discussed last, so feel free to skip the preamble if you’re able to deduce what I’m doing.
Note: I’ve only discussed accessors (getters) and not mutators (setters) today.
Some Reasonable Substitution Matrix Indexers
This is the notation you might expect from the indexer notation in C#.
// Let there be a class called SubMatrix which contains data from BLOSUM62. var sm = new SubMatrix( ... ); // I'll assume you already have some constructors. int index_of_proline = sm['P']; // Returns the row or column that corresponds to proline, 14. char token_at_three = sm[3]; // Returns the amino acid at position three, aspartate. int score_proline_to_aspartate = sm['P', 'D']; // Returns the score for a mutation from proline to aspartate, -1. int score_aspartate_to_proline = sm[3, 14]; // Returns the score for a mutation from aspartate to proline, -1.
An Example Bare Bones Substitution Matrix Class
Let’s say you’ve loaded up the BLOSUM62 and are representing it internally in some 2D array…
// We've keying the rows and columns in the order given by BLOSUM62: // ARNDCQEGHILKMFPSTWYVBZX* (24 rows, columns) int[,] imatrix;
For convenience, let’s say you’ll also keep a dictionary to map at which array position one finds each amino acid…
// Keys = amino acid letters, Values = row or column index Dictionary<char, int> indexOfAA;
Finally, we’ll put these two elements into a class and assume that you’ve already written your own constructors that will take care of the above two items — either from accepting arrays and strings as arguments or by reading from raw files. If this isn’t true and you need more help, feel free to leave a comment and I’ll extend this bare bones example.
// Bare bones class ... public partial class SubMatrix { // Properties ... private int[,] imatrix; private Dictionary<char, int> indexOfAA; // Automatic Properties ... public int Width { // Returns number of rows of the matrix. get { return imatrix.GetLength(0); } } // Constructors ... ... }
I’ve added the automatic property “Width” above — automatic properties are C# members that provide encapsulation: a public face to some arbitrary backend data — you’ve been using these all along when you’ve called “List.Count” or “Array.Length“.
Substitution Matrix Indexer Implementation
You can implement the example substitution matrix indexers as follows. Notice the use of the “this” keyword and square[] brackets[] to specify[] arguments[].
//And finally ... public partial class SubMatrix { // Indexers ... // Give me the row or column index where I can find the token "aa". public int this[char aa] { get { return this.indexOfAA[aa]; } } // Give me the amino acid at the row or column index "index". public char this[int index] { get { return this.key[index]; } } // Give me the score for mutating token "row" to token "column". public double this[char row, char column] { get { return this.imatrix[this.indexOfAA[row], this.indexOfAA[column]]; } } // Give me the score for mutating token at index "row" to index "column". public double this[int row, int column] { get { return this.imatrix[row, column]; } } }
Similar constructs are available in Python and Ruby but not Java. I’ll likely cover those later as well as how to set values too.